In this article, we’ll take a look at how to use native PowerShell cmdlets to download a file via HTTP, HTTPS, or FTP protocol. You can use PowerShell cmdlets as a native alternative of the wget and curl tool on Windows 10 and Windows Server 2016.
First, check the PowerShell version installed on your computer:
$psversiontable
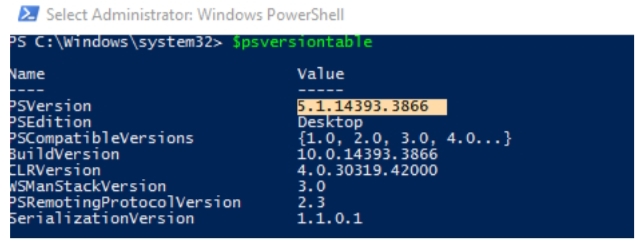
For versions of PowerShell earlier than 3.0, the System.Net.WebClient class must be used to download a file from the Internet.
On Windows 7/Windows Server 2008 R2 (on which PowerShell 2.0 is installed by default), you can use the following PowerShell commands to download a file from the HTTP(S) website and save it to a local drive:
$download_url = https://solutionviews.com
$local_path = “C:\PS\solutionviews_logo.png”
$WebClient = New-Object System.Net.WebClient
$WebClient.DownloadFile($download_url, $local_path)
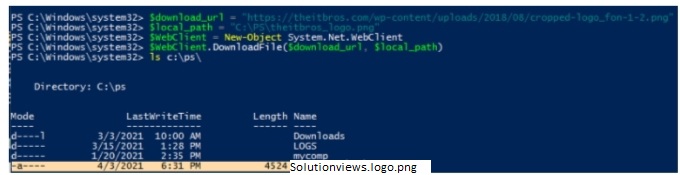
To download a file from an FTP server with authorization, you need to specify the FTP username and password in the script:
$download_url = ftp://hpe.com/iso/psp9.2.iso
$local_path = “C:\Downloads\psp9.2.iso “
$user = “hpeFtpUserName”
$pass = “Str0ng3$tPa$$”
$WebClient = New-Object System.Net.WebClient
$WebClient.Credentials = New-Object System.Net.NetworkCredential($user, $pass)
$WebClient.DownloadFile($download_url, $local_path)

On Windows 10, you can use the built-in Invoke-WebRequest cmdlet to download files (this cmdlet is available in all versions since PowerShell 3.0). To download a file, you just need to specify its URL and the local folder in which you want to save the file:
Invoke-WebRequest https://solutionviews.com-OutFile C:\ps\logo.png
Hint. By default, the Invoke-WebRequest cmdlet downloads the file to the current directory. If you need to change the directory and/or file name, use the –OutFile parameter.
On Windows 10, there are two aliases available for the Invoke-WebRequest cmdlet: curl and wget.
Shortcut command to download a file
to download a file from the Internet website, you can use a shorter command. Instead of typing a full cmdlet name, you can use (for example):
Wget https://solutionviews.com -OutFile C:\downloads\backup.zip
Hint. The Invoke-WebRequest doesn’t perform a check if the target file exists. If the file already exists, it is overwritten without any warning.
If you need to authenticate under the current user on a remote website (via NTLM or Kerberos), you need to add the –UseDefaultCredentials parameter:
Curl ‘http://theitbros.local/file.zip’ -UseDefaultCredentials
Or you can specify credentials manually:
Credentials = Get-Credential
Invoke-WebRequest -Uri https://solutionviews.com.com/backup_file.zip -OutFile C:\downloads\backup.zip -Credential $Credentials
Pass Additional Information
You can additionally pass some information in the HTTP header request. For example, set the API key:
Invoke-WebRequest -H @{‘apiKey’=’keyValue’} https://solutionviews.com/backup_file.zip -OutFile C:\ps\file.zip
Download the files
If you need to download a list of files in a batch, save their URLs in a plain text file and use the following command to start the download:
Get-Content urls.txt | ForEach-Object {Invoke-WebRequest $_ -OutFile $(Split-Path $_ -Leaf)}